public abstract class AbstractBaseAdapter<T> extends BaseAdapter { public LayoutInflater inflater; private Context context; public List<T> mList; protected int mInflaterId; BaseViewHolder baseViewHolder; public BaseViewHolder getBaseViewHolder() { if(this.baseViewHolder==null){ return null; } return baseViewHolder; } public void setBaseViewHolder(BaseViewHolder baseViewHolder) { this.baseViewHolder = baseViewHolder; } public AbstractBaseAdapter(Context context, List<T> mList, int mInflaterId) { this.context = context; this.mList = mList; this.mInflaterId = mInflaterId; inflater =LayoutInflater.from(context); } public AbstractBaseAdapter(Context context, List<T> mList, int mInflaterId, BaseViewHolder baseViewHolder) { this.context = context; this.mList = mList; this.mInflaterId = mInflaterId; this.baseViewHolder = baseViewHolder; } @Override public int getCount() { // TODO Auto-generated method stub return mList == null ? 0 : mList.size(); } @Override public T getItem(int position) { // TODO Auto-generated method stub return mList == null ? null : mList.get(position); } @Override public long getItemId(int position) { // TODO Auto-generated method stub return 0; } @Override public View getView(int position, View convertView, ViewGroup parent) { // TODO Auto-generated method stub BaseViewHolder holder = null; View view = convertView; if (view == null) { holder = baseViewHolder.getInstance(); view = inflater.inflate(mInflaterId, null); holder.bindView(view); view.setTag(holder); } else { holder = (BaseViewHolder) view.getTag(); } holder.setData(position); return view; } interface BaseViewHolder { /** * 得到Viewholder的实例 * * @return * @modifiedTime 下午4:: * @author lzt */ public BaseViewHolder getInstance(); /** * 绑定View * * @param v * @modifiedTime 下午4:: * @author lzt */ public void bindView(View v); /** * 设置数据 * * @param pos * @modifiedTime 下午4:: * @author lzt */ public void setData(int pos); }}package com.example.mylistview.adapter;import java.util.List;import android.content.Context;import android.view.View;import android.widget.CheckBox;import android.widget.TextView;import com.example.mylistview.R;import com.example.mylistview.ViewModel;public class MyBaseAdapter extends AbstractBaseAdapter { public MyBaseAdapter(Context context, List mList, int layoutId) { super(context, mList, layoutId); super.setBaseViewHolder(new ViewHolder()); } class ViewHolder implements BaseViewHolder { public TextView textView; public CheckBox checkBox; ViewHolder instance = null; @Override public BaseViewHolder getInstance() { // TODO Auto-generated method stub if (instance == null) { synchronized (ViewHolder.class) { if (instance == null) { instance = new ViewHolder(); } } } return instance; } @Override public void bindView(View v) { // TODO Auto-generated method stub textView = (TextView) v.findViewById(R.id.textView1); checkBox = (CheckBox) v.findViewById(R.id.checkBox1); } @Override public void setData(int pos) { textView.setText(((ViewModel) mList.get(pos)).getText()); checkBox.setChecked(((ViewModel) mList.get(pos)).isCheck()); } }}
推荐整理分享我的自定义Adapter(我的自定义计划怎么删除),希望有所帮助,仅作参考,欢迎阅读内容。
文章相关热门搜索词:我的自定义测试,我的自定义声音好了吗,我的自定义声音好了吗,我的自定义青年论文,我的自定义测试,我的自定义测试,我的自定义密码是多少,我的自定义青年,内容如对您有帮助,希望把文章链接给更多的朋友!
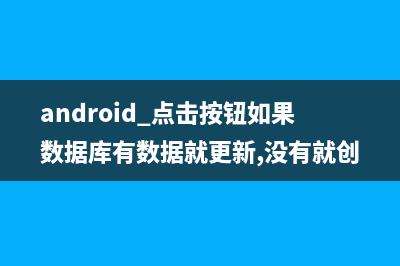
版权声明:本文为博主原创文章,未经博主允许不得转载。
Android 颜色对照表 颜色代码大全AA指定透明度。是完全透明。FF是完全不透明。超出取范围的将被恢复为默认。ffffffffffffffffffffccffffffffccffccffccffccffccccffccffff
Android 点击按钮隐藏键盘 直接上代码privatevoidcloseSoftInput(Contextcontext){if(context!=null){InputMethodManagerinputMethodManager=(InputMethodManager)context.getSystemService(Context.INPUT_METHOD_SERVICE);if(inputMetho
android framework 框架层功能梳理 转自: