#ifndef __HELLOWORLD_SCENE_H__ #define __HELLOWORLD_SCENE_H__ #include "cocos2d.h" using namespace cocos2d; class HelloWorld : public cocos2d::CCLayerColor { public: // Method 'init' in cocos2d-x returns bool, instead of 'id' in cocos2d-iphone (an object pointer) virtual bool init(); // there's no 'id' in cpp, so we recommend to return the class instance pointer static cocos2d::CCScene* scene(); // a selector callback void menuCloseCallback(CCObject* pSender); // preprocessor macro for "static create()" constructor ( node() deprecated ) CREATE_FUNC(HelloWorld); void addTarget(); void spriteMoveFinished(CCNode *sender); void gameLogic(cocos2d::CCTime dt); void ccTouchesEnded(CCSet *touches, CCEvent *event); CCArray *aarayTarget;//敌人 CCArray *arrayProjectile;//飞镖 void update(CCTime dt); }; #endif // __HELLOWORLD_SCENE_H__ HelloWorldScence.cpp[objc] view plaincopyprint?#include "HelloWorldScene.h" #include "SimpleAudioEngine.h" using namespace cocos2d; using namespace CocosDenshion; CCScene* HelloWorld::scene() { // 'scene' is an autorelease object CCScene *scene = CCScene::create(); // 'layer' is an autorelease object HelloWorld *layer = HelloWorld::create(); // add layer as a child to scene scene->addChild(layer); // return the scene return scene; } // on "init" you need to initialize your instance bool HelloWorld::init() { ////////////////////////////// // 1. super init first if ( CCLayerColor::initWithColor(ccc4(, , , )) ) { CCSize winSize = CCDirector::sharedDirector()->getWinSize();//获取屏幕大小 arrayProjectile = CCArray::create(); aarayTarget = CCArray::create(); float sprite_scale = 2.0; CCSprite *Player = CCSprite::create("Player.png"); Player->setScale(sprite_scale); Player->setPosition(ccp(Player->getContentSize().width*sprite_scale/2.0, winSize.height/2.0)); this->addChild(Player); aarayTarget->retain(); arrayProjectile->retain(); this->schedule(schedule_selector(HelloWorld::gameLogic), 1.0); this->schedule(schedule_selector(HelloWorld::update)); this->setTouchEnabled(true); return true; } else{ return false; } } void HelloWorld::gameLogic(cocos2d::CCTime dt){ this->addTarget(); } void HelloWorld::addTarget(){ CCSize winSize = CCDirector::sharedDirector()->getWinSize(); CCSprite *target = CCSprite::create("Target.png"); //随机位置 int minY = target->getContentSize().height/2.0; int maxY = winSize.height - target->getContentSize().height/2.0; int rangeY = maxY - minY; int actualY = rand()%rangeY minY; target->setPosition(ccp(winSize.width - target->getContentSize().width/2.0, actualY)); target->setTag(1); this->addChild(target); aarayTarget->addObject(target); //随机速度 float minDuration = 2.0; float maxDuration = 4.0; int rangeDuration = maxDuration - minDuration; float actualDuration = rand()%rangeDuration minDuration; CCFiniteTimeAction *actionMove = CCMoveTo::create(actualDuration, ccp(0 - target->getContentSize().width/2.0, actualY));//0代表屏幕外,这句表示在3秒内从初始位置移动到屏幕外 //增加一个回调函数,回收移动到屏幕外的精灵 CCFiniteTimeAction *actionMoveDone = CCCallFuncN::create(this, callfuncN_selector(HelloWorld::spriteMoveFinished)); target->runAction(CCSequence::create(actionMove,actionMoveDone,NULL)); } void HelloWorld::spriteMoveFinished(cocos2d::CCNode *sender){ CCSprite *sprite = (CCSprite *)sender; // this->removeChild(sprite, true); if (sprite->getTag() == 1) { aarayTarget->removeObject(sprite);//清除敌人 }else if(sprite->getTag() == 2){ arrayProjectile->removeObject(sprite);//清除飞镖 } } //发射飞镖 void HelloWorld::ccTouchesEnded(cocos2d::CCSet *touches, cocos2d::CCEvent *event){ CCTouch *touch = (CCTouch *)touches->anyObject(); CCPoint location = touch->getLocationInView(); location = this->convertTouchToNodeSpace(touch); CCSize winSize = CCDirector::sharedDirector()->getWinSize(); CCSprite *projectile = CCSprite::create("Projectile.png"); projectile->setPosition(ccp(, winSize.height/2)); float offX = location.x - projectile->getPositionX(); float offY = location.y - projectile->getPositionY(); if (offX <= 0) { return; } projectile->setTag(2); this->addChild(projectile); arrayProjectile->addObject(projectile); float angle = offY/offX; float realX= winSize.width projectile->getContentSize().width/2; float realY = realX * angle projectile->getPositionY(); CCPoint finalPosition = ccp(realX, realY); //获取飞镖飞行时间 float length = sqrtf(realX *realX realY*realY); float velocity = ; float realMoveDuration = length/velocity; projectile->runAction(CCSequence::create(CCMoveTo::create(realMoveDuration, finalPosition),CCCallFuncN::create(this, callfuncN_selector(HelloWorld::spriteMoveFinished)),NULL)); } //碰撞检测,消除飞镖和敌人 void HelloWorld::update(cocos2d::CCTime dt){ for (int i = 0; i < aarayTarget->count(); i) { CCSprite *target = (CCSprite *)aarayTarget->objectAtIndex(i); for (int j = 0; j < arrayProjectile->count(); j) { CCSprite *projectile = (CCSprite *)arrayProjectile->objectAtIndex(j); if (target->boundingBox().intersectsRect(projectile->boundingBox())) { aarayTarget->removeObjectAtIndex(i); arrayProjectile->removeObjectAtIndex(j); this->removeChild(target); this->removeChild(projectile); break; } } } } void HelloWorld::menuCloseCallback(CCObject* pSender) { CCDirector::sharedDirector()->end(); #if (CC_TARGET_PLATFORM == CC_PLATFORM_IOS) exit(0); #endif }
推荐整理分享Cocos2d-x碰撞检查与消灭的实现(cocos creator 碰撞检测),希望有所帮助,仅作参考,欢迎阅读内容。
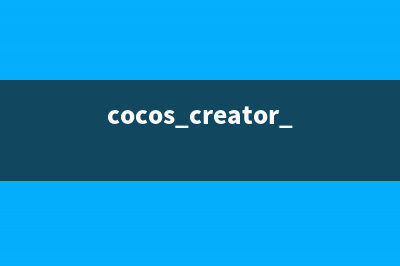
文章相关热门搜索词:cocoscreator碰撞,碰撞检测unity,cocos 碰撞,cocoscreator碰撞,cocos2dx碰撞检测,cocos 碰撞,cocos creator 碰撞检测,cocos2dx碰撞检测,内容如对您有帮助,希望把文章链接给更多的朋友!
非常好的cocos2d-x开发学习教程 COCOS2D开发者文档cocos2d,开发,IT,程序,教程,动画,游戏,文档,cocos2dxCOCOS2D-X开发者文档,一次下载不需要联网,所有资源单机,教程资源非常详细,包括:coc
在win7上python2.7环境下安装cocos-d-0.6.0 1.下载安装pyglet-1.2alpha1。由于我的系统已经安装python2.7,需自行安装pyglet下载地址:
OSX下cocos-2d的安装注意事项 1.同上篇win7中安装的基本步骤一样。先下载并安装pyglet1.2alpha版本。不过请使用root权限来安装。如果不清楚root密码,请按如下进行密码更改。MacBook-Air:~