#include <GLTools.h>#include <GLMatrixStack.h>#include <GLFrame.h>#include <GLFrustum.h>#include <GLBatch.h>#include <GLGeometryTransform.h>#include <math.h>#ifdef __APPLE__#include <glut/glut.h>#else#define FREEGLUT_STATIC#include <gl/glut.h>#endifGLShaderManager shaderManager; //着色管理器GLMatrixStack modelViewMatrix;GLMatrixStack projectionMatrix;GLFrame cameraFrame;GLFrame objectFrame;GLFrustum viewFrustum;GLTriangleBatch sphereBatch;GLTriangleBatch torusBatch;GLTriangleBatch cylinderBatch;GLTriangleBatch coneBatch;GLTriangleBatch diskBatch;GLGeometryTransform transformPipeline;M3DMatrixf shadowMatrix;GLfloat vGreen[] = { 0.0f, 1.0f, 0.0f, 1.0f };GLfloat vBlack[] = { 0.0f, 0.0f, 0.0f, 1.0f };int nStep = 0;void SetupRC(){glClearColor(0.7f, 0.7f, 0.7f, 1.0f);shaderManager.InitializeStockShaders(); 初始化glEnable(GL_DEPTH_TEST); // 开启深度测试transformPipeline.SetMatrixStacks(modelViewMatrix, projectionMatrix);cameraFrame.MoveForward(-.0f);gltMakeSphere(sphereBatch, 3.0, , );// 球体gltMakeTorus(torusBatch, 3.0f, 0.f, , ); // 花托gltMakeCylinder(cylinderBatch, 2.0f, 2.0f, 3.0f, , 2);//圆柱gltMakeCylinder(coneBatch, 2.0f, 0.0f, 3.0f, , 2);gltMakeDisk(diskBatch, 1.5f, 3.0f, , 3);//圆盘}void DrawWireFramedBatch(GLTriangleBatch* pBatch){shaderManager.UseStockShader(GLT_SHADER_FLAT, transformPipeline.GetModelViewProjectionMatrix(), vGreen);pBatch->Draw();// Draw black outlineglPolygonOffset(-1.0f, -1.0f);glEnable(GL_LINE_SMOOTH);glEnable(GL_BLEND);glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);glEnable(GL_POLYGON_OFFSET_LINE);glPolygonMode(GL_FRONT_AND_BACK, GL_LINE);glLineWidth(2.5f);shaderManager.UseStockShader(GLT_SHADER_FLAT, transformPipeline.GetModelViewProjectionMatrix(), vBlack);pBatch->Draw();// Restore polygon mode and depht testingglPolygonMode(GL_FRONT, GL_FILL);glDisable(GL_POLYGON_OFFSET_LINE);glLineWidth(1.0f);glDisable(GL_BLEND);glDisable(GL_LINE_SMOOTH);}void RenderScene(void){glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT | GL_STENCIL_BUFFER_BIT);modelViewMatrix.PushMatrix();M3DMatrixf mCamara;cameraFrame.GetCameraMatrix(mCamara);modelViewMatrix.MultMatrix(mCamara);M3DMatrixf mObjectFrame;objectFrame.GetMatrix(mObjectFrame);modelViewMatrix.MultMatrix(mObjectFrame);shaderManager.UseStockShader(GLT_SHADER_FLAT, transformPipeline.GetModelViewProjectionMatrix(), vBlack);switch (nStep) {case 0:DrawWireFramedBatch(&sphereBatch);break;case 1:DrawWireFramedBatch(&torusBatch);break;case 2:DrawWireFramedBatch(&cylinderBatch);break;case 3:DrawWireFramedBatch(&coneBatch);break;case 4:DrawWireFramedBatch(&diskBatch);break;}modelViewMatrix.PopMatrix();// Flush drawing commandsglutSwapBuffers();}// Respond to arrow keys by moving the camera frame of referencevoid SpecialKeys(int key, int x, int y){if (key == GLUT_KEY_UP)objectFrame.RotateWorld(m3dDegToRad(-5.0f), 1.0f, 0.0f, 0.0f);if (key == GLUT_KEY_DOWN)objectFrame.RotateWorld(m3dDegToRad(5.0f), 1.0f, 0.0f, 0.0f);if (key == GLUT_KEY_LEFT)objectFrame.RotateWorld(m3dDegToRad(-5.0f), 0.0f, 1.0f, 0.0f);if (key == GLUT_KEY_RIGHT)objectFrame.RotateWorld(m3dDegToRad(5.0f), 0.0f, 1.0f, 0.0f);glutPostRedisplay();}void KeyPressFunc(unsigned char key, int x, int y){if (key == ){nStep;if (nStep > 4)nStep = 0;}switch (nStep){case 0:glutSetWindowTitle("Sphere");break;case 1:glutSetWindowTitle("Torus");break;case 2:glutSetWindowTitle("Cylinder");break;case 3:glutSetWindowTitle("Cone");break;case 4:glutSetWindowTitle("Disk");break;}glutPostRedisplay();}void ChangeSize(int w, int h){glViewport(0, 0, w, h);viewFrustum.SetPerspective(.0f, float(w) / float(h), 1.0f, .0f);projectionMatrix.LoadMatrix(viewFrustum.GetProjectionMatrix());modelViewMatrix.LoadIdentity();}int main(int argc, char* argv[]){gltSetWorkingDirectory(argv[0]);glutInit(&argc, argv);glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGBA | GLUT_DEPTH | GLUT_STENCIL);glutInitWindowSize(, );glutCreateWindow("Sphere");glutReshapeFunc(ChangeSize);glutKeyboardFunc(KeyPressFunc);glutSpecialFunc(SpecialKeys);glutDisplayFunc(RenderScene);GLenum err = glewInit();if (GLEW_OK != err) {fprintf(stderr, "GLEW Error: %sn", glewGetErrorString(err));return 1;}SetupRC();glutMainLoop();return 0;}
推荐整理分享OpenGL 超级宝典学习,制作 圆柱,球体,花托,圆盘等多边体(opengl超级宝典pdf),希望有所帮助,仅作参考,欢迎阅读内容。
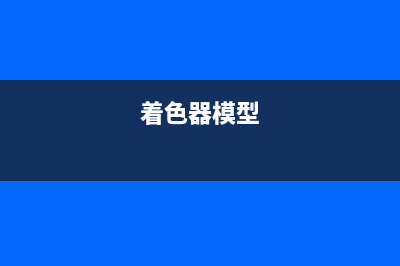
文章相关热门搜索词:opengl超级宝典第八版,opengl 超级宝典 编程指南,opengl超级宝典pdf 第七版百度云,opengl 超级宝典 第七版 pdf,opengl超级宝典pdf,opengl 超级宝典 编程指南,opengl超级宝典pdf,opengl超级宝典第七版,内容如对您有帮助,希望把文章链接给更多的朋友!
用球体模拟天空 之前说到可以用球体作为SkyDome模拟天空,那么就来说一说其中的细节.SkyDome就是天空穹顶,是一种在三维场景中模拟天空的方法,用SkyDome模拟的天空较SkyBox
如何向GLSL中传入多个纹理 如何向GLSL中传入多个纹理这几天在研究如何实现用GLSL对多个纹理进行融合处理,发现除了第一个纹理之外其它的纹理参数都无法传递到GLSL中去,在网
使用着色器模拟雾效果 上一篇关于天空盒的blog谈到了雾效果,那么这次来讨论一下用着色器实现雾效果的具体实现方法.雾在大自然中是一种常见的天气现象,比如清晨时分在山